Introduction
Having successfully installed Python on my Windows Mobile PDA I wanted to investigate if I could communicate between my Windows XP machine and my PDA using sockets. I used Cygwin to act as a Linux emulator, although there are Windows-based Python solutions, such as Enthought's Pylab.
Step One: Communication between processes on the same machine
As a first step, try communication between processes on a single machine.
I used the command ipconfig in a Windows command prompt to determine that my IP address is 192.168.1.64.
To create the server, I used the following Python code, which is largely from http://www.devshed.com/c/a/Python/Sockets-in-Python/1/.
Create a file called server.py with the following contents:
Then in a Python terminal running on a separate process type the following:
Step Two: Getting a simple Python script onto the PDA
Although it is possible to simply type commands at the command prompts, it can be difficult due to the input methods of the PDA and also it's prone to error. An easier method is to create the file and then copy it to the PDA.
To determine the default directory that Python works from type
>>> import os
>>> os.getcwd()
and in my case it was '\\Temp', as can be seen from the figure.
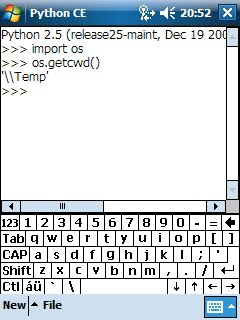
For example, create a simple script called test1.py that contains only one line
print 'Hello World'
Copy the file to the default directory and then type
>>> execfile('test1.py')
at the command prompt. You should then see the message displayed to the screen.
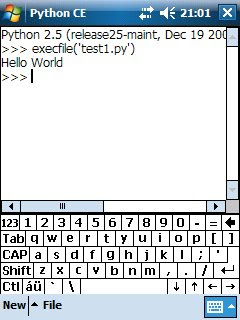
Step Three: Communication between a Windows machine and the Windows Mobile PDA
Create a file containing the following code:
>>> execfile('client.py')
Once it's been checked copy the file to a suitable directory on the PDA, e.g. in the Temp directory.
Running the client on the PDA you should then see a message sent to the server screen!
Having successfully installed Python on my Windows Mobile PDA I wanted to investigate if I could communicate between my Windows XP machine and my PDA using sockets. I used Cygwin to act as a Linux emulator, although there are Windows-based Python solutions, such as Enthought's Pylab.
Step One: Communication between processes on the same machine
As a first step, try communication between processes on a single machine.
I used the command ipconfig in a Windows command prompt to determine that my IP address is 192.168.1.64.
To create the server, I used the following Python code, which is largely from http://www.devshed.com/c/a/Python/Sockets-in-Python/1/.
Create a file called server.py with the following contents:
#! /usr/bin/env pythonTo make the Python file executable type chmod +x server.py at the command prompt.
import socket
mySocket = socket.socket (socket.AF_INET, socket.SOCK_STREAM)
mySocket.bind (( '192.168.1.64', 1200 ))
mySocket.listen (1)
while True:
channel, details = mySocket.accept()
print 'Opened a connection with', details
print channel.recv (100)
channel.send ( 'This is a message from the server.' )
channel.close()
Then in a Python terminal running on a separate process type the following:
Note that the value 47 is returned by the server and on requesting a message the server sends back 'This is a message from the server.' So this all worked fine.
>>> import socket
>>> mySocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
>>> mySocket.connect(('192.168.1.64', 1200))
>>> mySocket.send('Hello World. I have been sent from the client.')
47
>>> mySocket.recv(100)
'This is a message from the server.'
>>> mySocket.close()
Step Two: Getting a simple Python script onto the PDA
Although it is possible to simply type commands at the command prompts, it can be difficult due to the input methods of the PDA and also it's prone to error. An easier method is to create the file and then copy it to the PDA.
To determine the default directory that Python works from type
>>> import os
>>> os.getcwd()
and in my case it was '\\Temp', as can be seen from the figure.
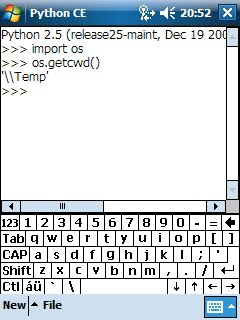
For example, create a simple script called test1.py that contains only one line
print 'Hello World'
Copy the file to the default directory and then type
>>> execfile('test1.py')
at the command prompt. You should then see the message displayed to the screen.
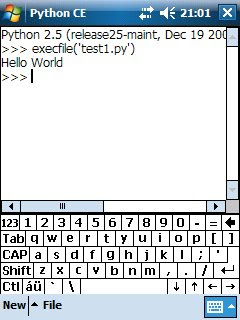
Step Three: Communication between a Windows machine and the Windows Mobile PDA
Create a file containing the following code:
import socket
mySocket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
mySocket.connect(('192.168.1.64', 1200))
mySocket.send('Hello World. I have been sent from the client.')
Save the code in the file client.py and check it by opening a terminal, starting Python and then typing>>> execfile('client.py')
Once it's been checked copy the file to a suitable directory on the PDA, e.g. in the Temp directory.
Running the client on the PDA you should then see a message sent to the server screen!
Comments